Introduction
In this post, I’ll be walking you through the process of adding a Javascript engine to Camunda. We’ll start by understanding the Camunda architecture and setting up a development environment. Next, I’ll guide you through writing the Javascript engine, integrating it with Camunda, and enhancing it. By the end of this post, you’ll have a good understanding of how to add a Javascript engine to Camunda.
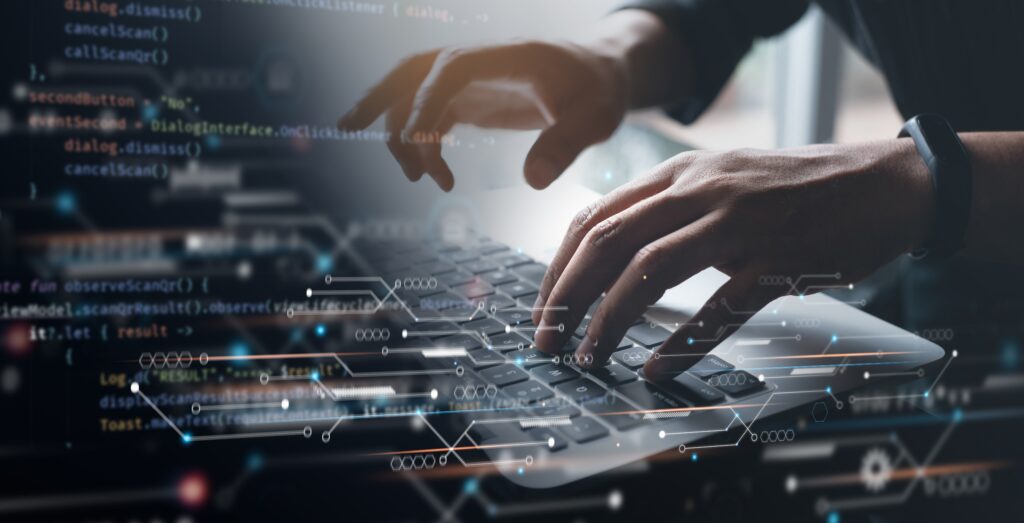
Setting up the Development Environment
Installing Camunda
To get started with Camunda, you’ll need to have a working installation. Camunda can be installed on various operating systems, including Windows, Mac, and Linux. There are several ways to install Camunda, including using a Docker container, installing it locally, or deploying it to a cloud platform. You can find detailed instructions for each installation method on the Camunda website.
Understanding the Camunda Architecture
To understand how to add a Javascript engine to Camunda, it’s important to understand the architecture of the platform. Camunda is built on a modular architecture that allows you to add custom components and extend the platform’s capabilities. The core components of Camunda include the Camunda Modeler, the Camunda Engine, and the Camunda REST API. The Camunda Modeler is used for designing workflows, the Camunda Engine is responsible for executing workflows, and the Camunda REST API is used for communicating with the Camunda Engine.
Setting up a Development Workspace
To add a Javascript engine to Camunda, you’ll need a development workspace. You can set up a development workspace using any code editor or Integrated Development Environment (IDE) of your choice. Some popular options include Visual Studio Code, IntelliJ, and Eclipse. I recommend using Visual Studio Code for this project, as it is a lightweight and powerful code editor that is well suited for developing Camunda applications.
Importing the Camunda BPM Library
Camunda provides a Java library that can be used to interact with the Camunda Engine. You’ll need to import this library into your development workspace in order to add a Javascript engine to Camunda. The library can be found on the Camunda website, and you can use a build management tool like Maven or Gradle to import it into your project.
Setting up a Camunda Project
Now that you have a development workspace set up and the Camunda BPM library imported, you can set up a new Camunda project. To do this, create a new Java project in your development workspace and import the Camunda BPM library. You should also create a new class that will be used to implement your Javascript engine.
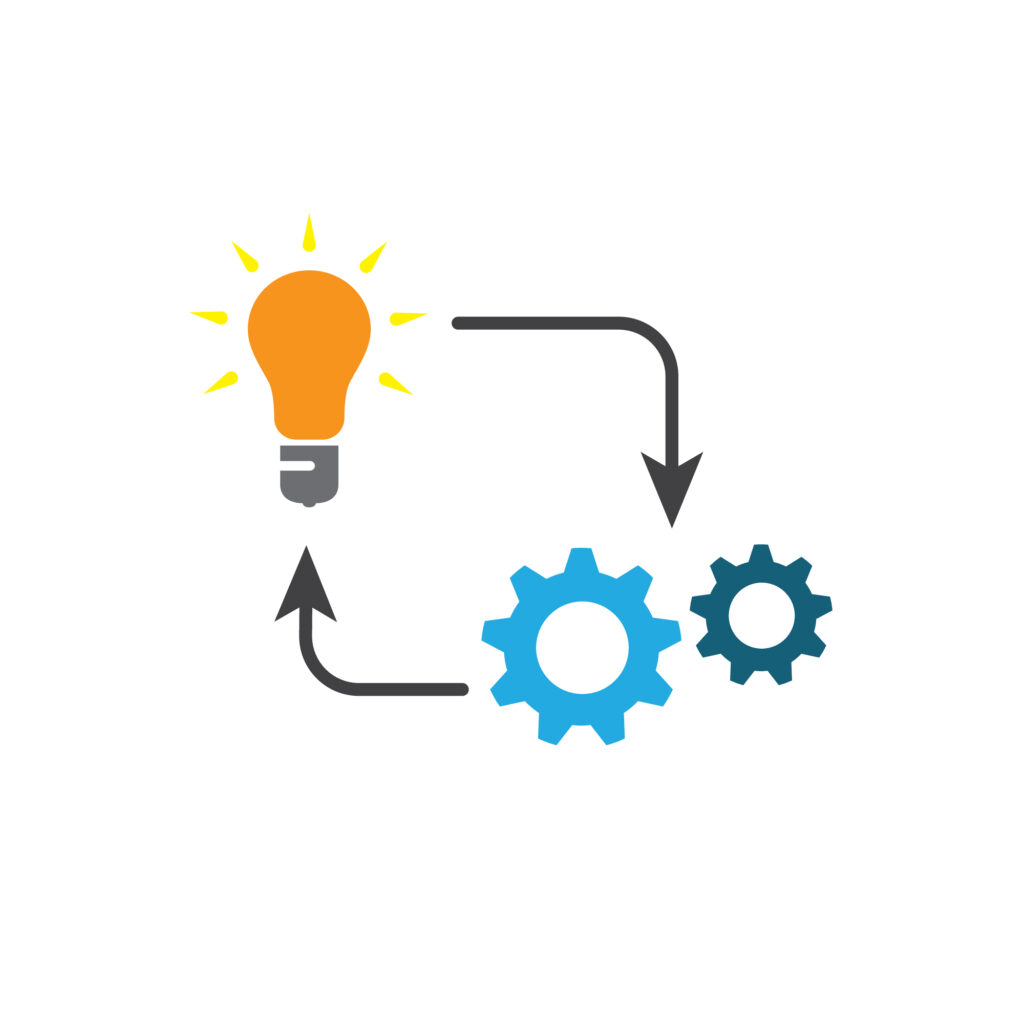
Implementing the JavaScript Engine in Camunda
Once you have set up your environment and have your JavaScript code ready, it’s time to integrate it into Camunda. This process will involve a few steps:
Register the JavaScript Engine
The first step is to register the JavaScript engine with Camunda. You can do this by creating a custom plugin that extends the Camunda engine. This plugin should implement the org.camunda.bpm.engine.impl.scripting.ScriptEngine service and declare the JavaScript engine as its implementation. You can then register this plugin in the Camunda engine configuration file.
Create a Custom Task
Next, you need to create a custom task that will use your JavaScript engine. To do this, you will need to extend the org.camunda.bpm.engine.delegate.JavaDelegate class and implement the execute() method. In this method, you can retrieve the JavaScript code from your script file and execute it using the registered JavaScript engine.
Deploy the Process Definition
Finally, you need to deploy the process definition that includes your custom task. You can do this by creating a BPMN process definition file and deploying it to the Camunda engine using the Camunda REST API or the Camunda Tasklist.
Once you have completed these steps, you can test your JavaScript engine in Camunda by starting a process instance and checking the output of your custom task. If everything is working correctly, you should see the output of your JavaScript code in the Camunda Tasklist or the Camunda Cockpit.
In conclusion, adding a JavaScript engine to Camunda is a straightforward process that can greatly enhance the functionality of your Camunda BPMN processes. Whether you need to access external APIs, perform complex calculations, or interact with other systems, integrating a JavaScript engine into Camunda can help you achieve your goals. With a little bit of setup and implementation, you can start using JavaScript in Camunda today!
Deploying the JavaScript Engine in Camunda
Once we have implemented the JavaScript engine in Camunda, we need to deploy it in the Camunda environment. Here are the steps to do that:
- Copy the compiled JavaScript engine jar file to the Camunda lib directory.
- Add the engine configuration to the Camunda configuration file (camunda.cfg.xml). The configuration file should include the following properties:
- Engine name
- Engine class
- Engine configuration URL
- Engine configuration file name
- Restart the Camunda engine after adding the engine configuration to the configuration file.
- Test the JavaScript engine by executing a BPMN process that has a script task that uses the JavaScript engine.
- If the JavaScript engine is working as expected, we can deploy it to a production environment.
- To deploy to a production environment, we need to perform the same steps as in a development environment, but with additional security and performance considerations in mind.
In conclusion, adding a JavaScript engine to Camunda is a straightforward process that involves writing the JavaScript engine code, compiling it, deploying it in the Camunda environment, and testing it. With a few modifications, we can use the same JavaScript engine for multiple Camunda projects, saving time and effort in the long run.
Testing and Debugging the JavaScript Engine in Camunda
After deploying the JavaScript engine in Camunda, we need to test it to ensure that it is working as expected. Here are the steps to test and debug the JavaScript engine:
- Execute a BPMN process that has a script task that uses the JavaScript engine.
- Verify that the script task is executed correctly and produces the expected output.
- If the script task does not produce the expected output, we need to debug the JavaScript engine code.
- Camunda provides a debugging interface in the Camunda Tasklist that allows us to inspect the script task execution, variables, and outputs. We can use this interface to debug the JavaScript engine.
- If we are unable to resolve the issue with the Camunda Tasklist debugging interface, we can also use the Camunda Cockpit to inspect the script task execution, variables, and outputs.
- In addition to the Camunda debugging interfaces, we can also use a standalone JavaScript debugger, such as the Chrome DevTools or Firebug, to debug the JavaScript engine.
- If the issue still persists after using the Camunda debugging interfaces and a standalone JavaScript debugger, we can log the execution of the script task and the values of the variables and outputs.
- Once the issue is resolved, we need to repeat the testing process to verify that the JavaScript engine is working correctly.
In conclusion, testing and debugging the JavaScript engine in Camunda is a critical step in ensuring that the engine is working as expected. Camunda provides several debugging interfaces and tools that allow us to debug the JavaScript engine effectively. Additionally, logging the execution of the script task can also help us identify and resolve issues with the JavaScript engine.
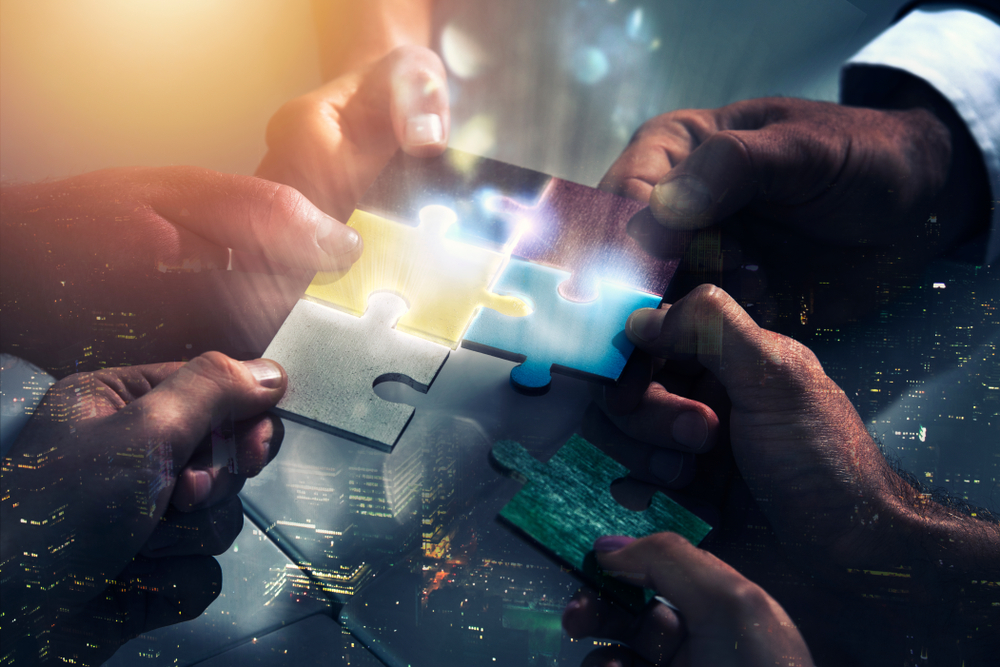
Integrating JavaScript with Camunda
In this part, I’ll walk you through the process of integrating your custom JavaScript engine with Camunda. This integration is crucial to make sure that Camunda can execute your JavaScript expressions correctly and the correct runtime environment is used.
To integrate your JavaScript engine with Camunda, you need to implement the org.camunda.bpm.engine.impl.scripting.ScriptEngine interface provided by Camunda. This interface requires you to implement a few methods such as eval, execute, setScriptEngineFactory, and unsetScriptEngineFactory.
The eval method is used to evaluate a single JavaScript expression and return the result. The execute method is used to execute a full JavaScript script, which may contain multiple statements. Both of these methods should return the result of the JavaScript expression or script.
The setScriptEngineFactory method is used to set the org.camunda.bpm.engine.impl.scripting.ScriptEngineFactory for your custom JavaScript engine. This factory is responsible for creating instances of your custom JavaScript engine for use in Camunda. The unsetScriptEngineFactory method is used to unset the script engine factory and remove it from Camunda.
Once you have implemented the ScriptEngine interface, you need to register it with Camunda. To do this, you can add a file named org.camunda.bpm.engine.impl.scripting.ScriptEngine to the META-INF/services directory in your classpath. This file should contain the fully-qualified name of your custom JavaScript engine class.
At this point, your custom JavaScript engine should be fully integrated with Camunda and ready to use. To test the integration, you can create a process definition that uses JavaScript expressions and verify that they are executed correctly by your custom JavaScript engine.
In conclusion, integrating a custom JavaScript engine with Camunda is a straightforward process that involves implementing the ScriptEngine interface and registering it with Camunda. With this integration in place, you can use your custom JavaScript engine in your Camunda processes and take advantage of the powerful scripting capabilities that it provides.
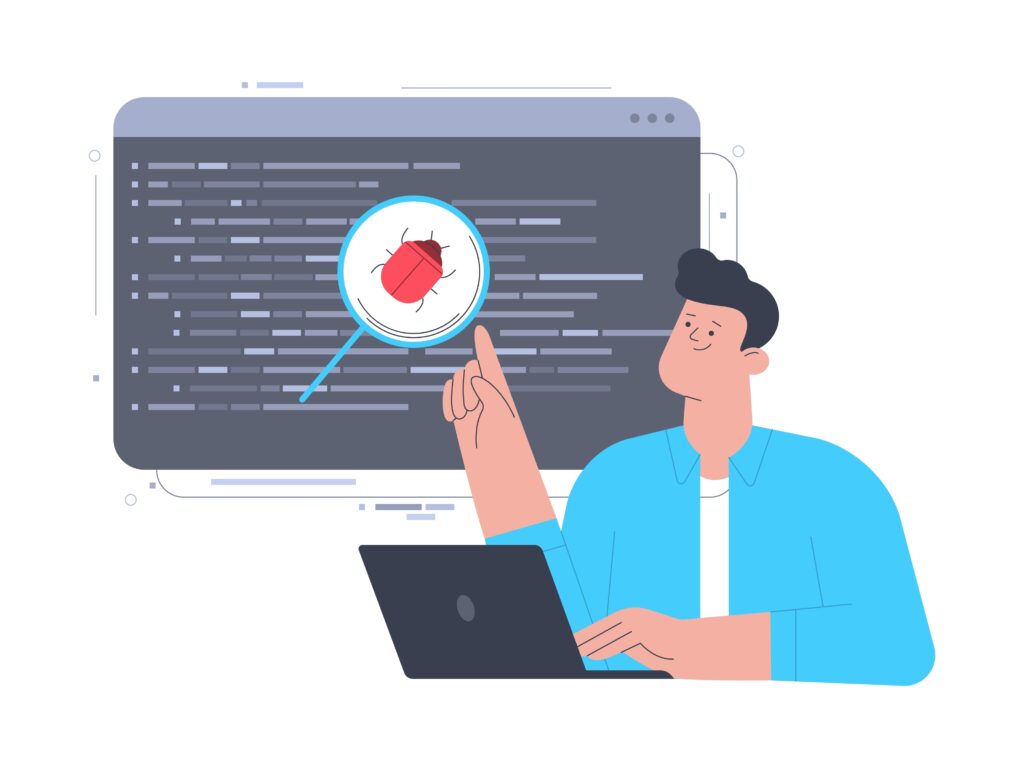
Testing and Debugging Your JavaScript Engine
Once you have completed all the previous steps, it’s time to test your JavaScript engine to make sure everything is working as expected. Before you start testing, it’s important to have a clear understanding of what you want to achieve with your engine and what kind of behavior you expect from it. This will help you to determine whether your implementation is correct or if there are any bugs that need to be fixed.
There are several ways to test a JavaScript engine, including manual testing and automated testing. In manual testing, you will execute your engine in a test environment and observe its behavior. This approach is useful when you need to test specific scenarios or edge cases. On the other hand, automated testing is more efficient and less prone to human error. You can write test cases that will execute your engine automatically and check whether it produces the expected results.
Debugging is another important step in testing your JavaScript engine. If you encounter any issues or errors, you need to be able to diagnose the root cause and fix the problem. One way to debug your engine is to use the built-in debugger in your development environment. You can set breakpoints, inspect variables, and step through your code to see what’s happening at each stage.
Another way to debug your engine is to log messages to the console. By logging messages, you can see what’s happening inside your engine and diagnose any issues. This approach is particularly useful when you’re working with complex systems or when you need to track down hard-to-find bugs.
In conclusion, testing and debugging your JavaScript engine is a critical step in the process of adding it to Camunda. By testing your engine, you can ensure that it works as expected and that it produces the desired results. Debugging is also important to help you identify and fix any issues that may arise during the testing process. With these tools and techniques at your disposal, you can make sure that your JavaScript engine is fully functional and ready to be used in your Camunda applications.